Fine-Tuning a Hugging Face Model: A Comprehensive Guide
- Bizzsoft Digital
- Jan 11
- 3 min read
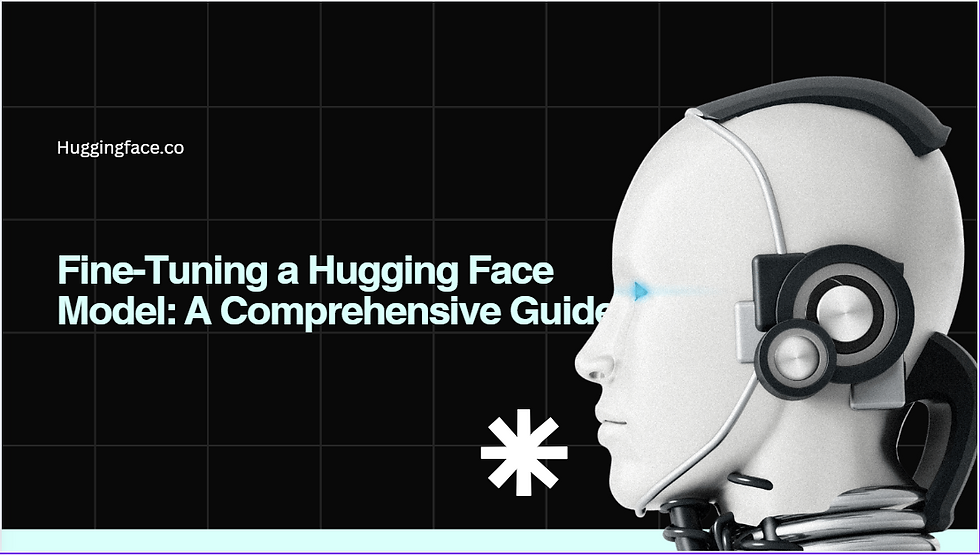
This article provides a detailed walkthrough of how to fine-tune a pre-trained model from Hugging Face's transformers library. We'll cover the essential steps involved, from preparing your dataset to evaluating the model's performance. By the end of this guide, you'll be equipped to fine-tune a model for a variety of natural language processing tasks such as text classification, summarization, or named entity recognition.
1. Setting up the Environment
Before we begin, ensure you have the necessary libraries installed. You can install them using pip:
pip install transformers datasets
Optionally, install accelerate if you plan to leverage multi-GPU or distributed training:
pip install accelerate
2. Choosing a Model and Dataset
Model Selection
Hugging Face offers a vast collection of pre-trained models for various tasks such as text classification, question answering, and language generation. Select a model appropriate for your needs. For this example, we'll use bert-base-uncased, a commonly used model for text classification tasks.
Dataset Preparation
You can either use a dataset from the Hugging Face datasets library or create your own. For this tutorial, we'll use the sst2 dataset, a sentiment classification dataset.
from datasets import load_dataset
dataset = load_dataset('sst2')
3. Preprocessing the Data
Tokenization
Tokenization is the process of breaking down text into smaller units (tokens). For this step, we use the tokenizer corresponding to the pre-trained model.
from transformers import AutoTokenizer
tokenizer = AutoTokenizer.from_pretrained('bert-base-uncased')
def preprocess_function(examples):
return tokenizer(examples['sentence'], padding="max_length", truncation=True)
encoded_dataset = dataset.map(preprocess_function, batched=True)
This code tokenizes the sentence column of the dataset, truncating text to the model’s maximum input size and applying padding.
Dataset Splits
Ensure the dataset is split into training, validation, and test sets. If using a custom dataset, split it manually.
train_dataset = encoded_dataset['train']
val_dataset = encoded_dataset['validation']
test_dataset = encoded_dataset['test']
4. Fine-Tuning with the Trainer API
The Hugging Face Trainer API simplifies the fine-tuning process, allowing you to focus on hyperparameters and results.
Training Arguments
Define the training parameters such as learning rate, batch size, number of epochs, and output directory.
from transformers import TrainingArguments
training_args = TrainingArguments(
output_dir="./results",
learning_rate=2e-5,
per_device_train_batch_size=16,
per_device_eval_batch_size=16,
num_train_epochs=3,
weight_decay=0.01,
evaluation_strategy="epoch",
save_strategy="epoch",
logging_dir="./logs",
logging_steps=10,
)
Metrics for Evaluation
Define a function to compute evaluation metrics. Here, we use accuracy as an example.
import numpy as np
from datasets import load_metric
metric = load_metric("accuracy")
def compute_metrics(pred):
labels = pred.label_ids
preds = pred.predictions.argmax(-1)
return metric.compute(predictions=preds, references=labels)
Trainer Setup
Instantiate the Trainer with the model, training arguments, datasets, and metric function.
from transformers import AutoModelForSequenceClassification, Trainer
model = AutoModelForSequenceClassification.from_pretrained('bert-base-uncased', num_labels=2)
trainer = Trainer(
model=model,
args=training_args,
train_dataset=train_dataset,
eval_dataset=val_dataset,
compute_metrics=compute_metrics,
)
Training
Start the fine-tuning process.
trainer.train()
5. Evaluation
Evaluate the model’s performance on the test set.
eval_results = trainer.evaluate(test_dataset)
print(f"Evaluation results: {eval_results}")
6. Saving the Model
Save the fine-tuned model and tokenizer for later use.
trainer.save_model("./fine_tuned_model")
tokenizer.save_pretrained("./fine_tuned_model")
7. Deploying the Model
You can now deploy the fine-tuned model using Hugging Face's model hub, or integrate it into your applications for inference.
Pushing to Hugging Face Hub
model.push_to_hub("your_model_name")
tokenizer.push_to_hub("your_model_name")
Using for Inference
from transformers import pipeline
classifier = pipeline("text-classification", model="./fine_tuned_model")
result = classifier("This is a great movie!")
print(result)
Additional Tips
Experiment with Hyperparameters: Fine-tuning often requires experimenting with learning rates, batch sizes, and the number of epochs.
Early Stopping: Implement early stopping to prevent overfitting, especially on smaller datasets.
Data Augmentation: Use data augmentation techniques if your dataset is small to enhance model generalization.
Monitor Training: Use tools like TensorBoard to visualize metrics and monitor the training process.
Distributed Training: Utilize accelerate for scaling up to multiple GPUs or distributed environments.
This comprehensive guide equips you with the knowledge and tools to successfully fine-tune a Hugging Face model. By following these steps, you can adapt pre-trained models to your specific use cases, achieving state-of-the-art performance for a variety of NLP tasks. Happy fine-tuning!
Kommentare