Integrating Hugging Face AI Models into Your Python Applications: A Comprehensive Guide
- Bizzsoft Digital
- Jan 11
- 6 min read
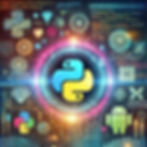
Hugging Face has revolutionized the field of artificial intelligence by providing accessible, state-of-the-art models for various tasks, including natural language processing (NLP), computer vision, and more. This guide will walk you through the process of downloading these models to your local machine and integrating them into your Python applications.
Prerequisites:
Before you begin, ensure your system meets the following requirements:
Python: Install Python version 3.6 or higher. While versions 3.8 or 3.9 are often recommended for compatibility, the latest version should also work. citeturn0search5
pip: Ensure that pip, Python's package installer, is installed and updated.
Virtual Environment: It's advisable to create a virtual environment to manage your project's dependencies and avoid conflicts.
python -m venv myenv
source myenv/bin/activate # For Linux/macOS
myenv\Scripts\activate    # For Windows
```
Â
Text Editor or IDE: Use a code editor like Visual Studio Code, PyCharm, or Jupyter Notebook for writing and running your Python scripts.
Step 1: Install the Transformers Library
The Hugging Face Transformers library is essential for accessing and utilizing pre- trained models. Install it using pip:
pip install transformers
```
Â
If you plan to leverage GPU acceleration for improved performance, ensure that you have either PyTorch or TensorFlow installed, depending on your preference:
Â
```bash
pip install torch torchvision torchaudio # For PyTorch
# or
pip install tensorflow                  # For TensorFlow
```
Â
---
Â
Step 2: Choose and Download a Model
Â
Hugging Face offers a vast repository of pre-trained models suitable for various tasks. You can browse the [Hugging Face Model Hub](https://huggingface.co/models) to select a model that fits your application's requirements. Each model page provides detailed information, including the model's intended use cases, architecture, and performance metrics.
Â
To download and initialize a model, you can use the `pipeline` function, which simplifies the process by handling both the model and tokenizer:
Â
```python
from transformers import pipeline
Â
# Initialize a sentiment-analysis pipeline
classifier = pipeline("sentiment-analysis")
Â
# Use the classifier
result = classifier("I love using Hugging Face models!")
print(result)
```
Â
For more control over the model and tokenizer, you can use the `AutoModel` and `AutoTokenizer` classes:
Â
```python
from transformers import AutoModelForSequenceClassification, AutoTokenizer
Â
model_name = "bert-base-uncased"Â # Specify the model name
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModelForSequenceClassification.from_pretrained(model_name)
```
Â
---
Â
Step 3: Utilize the Model in Your Application
Â
Once you've loaded the model and tokenizer, you can integrate them into your application. Here's an example of performing sentiment analysis:
Â
```python
from transformers import pipeline
Â
# Initialize the sentiment-analysis pipeline
classifier = pipeline("sentiment-analysis")
Â
# Analyze multiple texts
texts = ["This is a fantastic product!", "I'm not satisfied with the service."]
results = classifier(texts)
Â
# Display the results
for text, result in zip(texts, results):
   print(f"Text: {text}\nSentiment: {result['label']} (Confidence: {result['score']:.2f})\n")
```
---
Â
Example Project: Text Summarization Application
Â
Let's build a simple text summarization tool using a pre-trained model from Hugging Face:
Â
```python
from transformers import pipeline
Â
# Initialize the summarization pipeline
summarizer = pipeline("summarization")
Â
def summarize_text(text):
   """Summarizes the input text."""
   summary = summarizer(text, max_length=130, min_length=30, do_sample=False)
   return summary[0]['summary_text']
Â
if name == "__main__":
   # Sample text
   article = """
   Artificial intelligence (AI) is transforming industries by automating tasks, providing insights through data
Â
Â
```
Â
---
Â
Step 2: Choose and Download a Model
Â
Hugging Face offers a vast repository of pre-trained models suitable for various tasks. You can browse the [Hugging Face Model Hub](https://huggingface.co/models) to select a model that fits your application's requirements. Each model page provides detailed information, including the model's intended use cases, architecture, and performance metrics.
Â
To download and initialize a model, you can use the `pipeline` function, which simplifies the process by handling both the model and tokenizer:
Â
```python
from transformers import pipeline
Â
# Initialize a sentiment-analysis pipeline
classifier = pipeline("sentiment-analysis")
Â
# Use the classifier
result = classifier("I love using Hugging Face models!")
print(result)
```
Â
For more control over the model and tokenizer, you can use the `AutoModel` and `AutoTokenizer` classes:
Â
```python
from transformers import AutoModelForSequenceClassification, AutoTokenizer
Â
model_name = "bert-base-uncased"Â # Specify the model name
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModelForSequenceClassification.from_pretrained(model_name)
```
Â
---
Â
Step 3: Utilize the Model in Your Application
Â
Once you've loaded the model and tokenizer, you can integrate them into your application. Here's an example of performing sentiment analysis:
Â
```python
from transformers import pipeline
Â
# Initialize the sentiment-analysis pipeline
classifier = pipeline("sentiment-analysis")
Â
# Analyze multiple texts
texts = ["This is a fantastic product!", "I'm not satisfied with the service."]
results = classifier(texts)
Â
# Display the results
for text, result in zip(texts, results):
   print(f"Text: {text}\nSentiment: {result['label']} (Confidence: {result['score']:.2f})\n")
```
Â
---
Â
Do's and Don'ts
Â
Do’s:
Â
Understand the Model: Read the model's documentation to grasp its capabilities and limitations. This understanding is crucial for effective application. citeturn0search22
Â
Experiment: Test multiple models to determine which one best suits your specific task. The Hugging Face Model Hub offers a variety of models for different applications.
Â
Optimize Performance: Consider using GPU acceleration and batch processing to enhance performance, especially when handling large datasets.
Â
Don't:
Â
Assume Perfection: Pre-trained models may have biases or limitations based on their training data. Always validate and, if necessary, fine-tune models to align with your specific requirements.
Â
Ignore Licensing: Adhere to the licensing terms of the models you use, and provide appropriate attribution when deploying them in your applications.
Â
---
Â
Advanced Tips
Â
1. Fine-Tuning: To achieve better performance on specific tasks, consider fine-tuning a pre-trained model with your dataset. Hugging Face provides comprehensive tutorials on how to fine-tune models for various tasks. citeturn0search2
Â
2. GPU Acceleration: Leveraging GPUs can significantly speed up model inference and training. Ensure that your system has the necessary hardware and that you've installed compatible versions of deep learning frameworks like PyTorch or TensorFlow.
Â
3. Caching: Hugging Face models are cached locally after the first download, reducing the need for repeated downloads and enabling faster initialization in future uses.
Â
4. Community Engagement: Join the Hugging Face community forums and GitHub repositories to stay updated, seek support, and contribute to ongoing developments.
Â
---
Â
Example Project: Text Summarization Application
Â
Let's build a simple text summarization tool using a pre-trained model from Hugging Face:
Â
from transformers import pipeline
# Initialize summarizer
summarizer = pipeline("summarization")
def summarize_text(text):
summary = summarizer(text, max_length=150, min_length=40, do_sample=False)
return summary[0]["summary_text"]
if __name__ == "__main__":
long_text = """
Artificial Intelligence is transforming industries with advanced tools that allow
machines to learn, adapt, and perform human-like tasks. AI applications are
widespread, from healthcare diagnostics to self-driving cars.
"""
print("Original Text:", long_text)
print("\nSummary:", summarize_text(long_text))
from transformers import pipeline
# Initialize NER pipeline
ner = pipeline("ner", grouped_entities=True)
text = "Hugging Face Inc. is based in New York City and specializes in NLP technologies."
# Perform Named Entity Recognition
entities = ner(text)
print(entities)
 Common Challenges and Solutions
Model Size and Storage: Large models like GPT-3 require significant storage and memory. Use optimized, distilled versions (e.g., distilBERT) if resources are limited.
Latency in Inference: Use batching for inference or switch to faster hardware (e.g., GPUs or TPUs) for production environments.
Ethical and Bias Concerns: Models may exhibit biases present in their training data. Always validate outputs, especially for sensitive applications.
Compatibility Issues: Ensure that library versions (e.g., Transformers, PyTorch) are compatible with each other. Use:
bash
Copy code
pip list | grep transformers pip list | grep torch
Real-World Use Cases
Chatbots: Build conversational agents using models like DialoGPTÂ or ChatGPT.
Recommendation Systems: Use embeddings from models like BERTÂ to create personalized recommendations.
Language Translation: Utilize models like mBARTÂ for multilingual applications.
Sentiment Analysis for Market Research: Monitor customer sentiment on social media using sentiment analysis pipelines.
Conclusion
Hugging Face provides an intuitive and powerful platform for integrating AI into your Python applications. Whether you're building a chatbot, conducting sentiment analysis, or creating custom NLP pipelines, Hugging Face models simplify development while delivering state-of-the-art performance. By following the steps and tips in this guide, you can confidently navigate the world of AI and create impactful, efficient applications.
For more resources, check out the Hugging Face documentation. Explore the community forums for insights, tips, and collaborations. The possibilities with Hugging Face are endless—get started today!